- A+
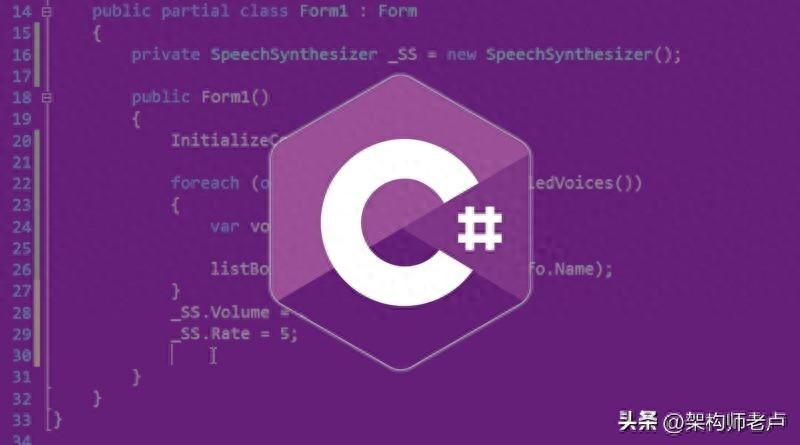
概述:C#中的Attribute(特性)为程序元素提供了灵活的元数据机制。除基础应用外,可高级应用于自定义代码生成、AOP等领域。通过示例展示了Attribute在AOP中的实际用途,以及如何通过反射机制获取并执行与Attribute相关的逻辑。
在C#中,Attribute(特性)是一种用于为程序实体(如类、方法、属性等)添加元数据的机制。它们提供了一种在运行时向程序元素添加信息的灵活方式。Attribute通常用于提供关于程序元素的附加信息,这些信息可以在运行时被反射(reflection)机制访问。
功用和作用:
- 元数据添加: Attribute允许程序员向代码添加元数据,这些元数据提供关于程序元素的额外信息。
- 运行时信息获取: 通过反射,可以在运行时检索Attribute,从而动态获取与程序元素相关的信息。
- 代码分析: Attribute可以用于代码分析工具,使其能够更好地理解和处理代码。
应用场景:
- 序列化: 在进行对象序列化时,可以使用Attribute指定序列化的方式。
- ASP.NET MVC: 在MVC框架中,Attribute用于指定路由、行为等信息。
- 单元测试: Attribute可用于标记测试方法,提供测试框架更多的信息。
- 安全性: Attribute可以用于标记一些安全相关的信息,如权限控制。
提供方法及步骤:
下面通过一个简单的例子来演示在C#中使用Attribute的方法和步骤。我们将创建一个自定义Attribute,然后将其应用于一个类的属性上。
using System; // 定义一个自定义Attribute [AttributeUsage(AttributeTargets.Property, Inherited = false, AllowMultiple = false)] sealed class MyCustomAttribute : Attribute { public string Description { get; } public MyCustomAttribute(string description) { Description = description; } } // 应用Attribute的类 class MyClass { // 应用自定义Attribute到属性上 [MyCustomAttribute("This is a custom attribute.")] public string MyProperty { get; set; } } class Program { static void Main() { // 使用反射获取Attribute信息 var property = typeof(MyClass).GetProperty("MyProperty"); var attribute = (MyCustomAttribute)Attribute.GetCustomAttribute(property, typeof(MyCustomAttribute)); // 输出Attribute的信息 if (attribute != null) { Console.WriteLine($"Attribute Description: {attribute.Description}"); } else { Console.WriteLine("Attribute not found."); } } }
在这个例子中,我们创建了一个名为MyCustomAttribute的自定义Attribute,并将其应用于MyClass类的MyProperty属性。然后,在Main方法中,我们使用反射获取并输出Attribute的信息。
C#的Attribute可以用于更复杂的场景
例如:
- 自定义代码生成: 通过在Attribute中添加代码生成的逻辑,可以在编译时生成额外的代码。这在某些框架中是常见的做法,比如ASP.NET MVC中的一些Attribute可以生成路由映射代码。
- AOP(面向切面编程): Attribute可以用于实现AOP,通过在方法上添加Attribute来定义切面逻辑,如日志记录、性能监控等。
- 自定义序列化/反序列化: 可以使用Attribute来定义对象序列化和反序列化的方式,以满足特定的需求。
- ORM(对象关系映射): 一些ORM框架使用Attribute来映射类和数据库表之间的关系,以及属性和表字段之间的对应关系。
下面通过一个简单的例子来演示AOP的应用,其中使用Attribute实现一个简单的日志记录:
using System; [AttributeUsage(AttributeTargets.Method, Inherited = false, AllowMultiple = false)] sealed class LogAttribute : Attribute { public void BeforeCall() { Console.WriteLine("Method execution started at: " + DateTime.Now); } public void AfterCall() { Console.WriteLine("Method execution completed at: " + DateTime.Now); } } class Example { [Log] public void MyMethod() { Console.WriteLine("Executing the method..."); } } class Program { static void Main() { var example = new Example(); var method = typeof(Example).GetMethod("MyMethod"); // 使用反射获取Attribute并执行相应逻辑 var logAttribute = (LogAttribute)Attribute.GetCustomAttribute(method, typeof(LogAttribute)); if (logAttribute != null) { logAttribute.BeforeCall(); } // 调用方法 example.MyMethod(); if (logAttribute != null) { logAttribute.AfterCall(); } } }
运行效果:
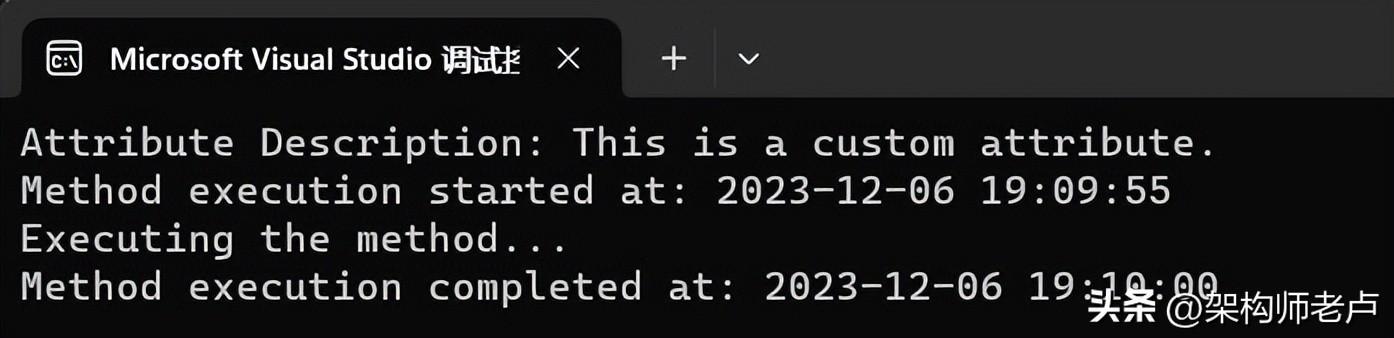
在这个例子中,我们定义了一个LogAttribute,它包含了在方法执行前后记录日志的逻辑。然后,我们在MyMethod方法上应用了这个Attribute。在Main方法中,使用反射获取Attribute并执行相应的逻辑,从而实现了在方法执行前后记录日志的功能。
这是一个简单的AOP例子,实际应用中可以根据需求定义更复杂的Attribute和逻辑。
源代码获取:https://pan.baidu.com/s/1OCKBCzTpgmI6Bdb_24M1BQ?pwd=6666